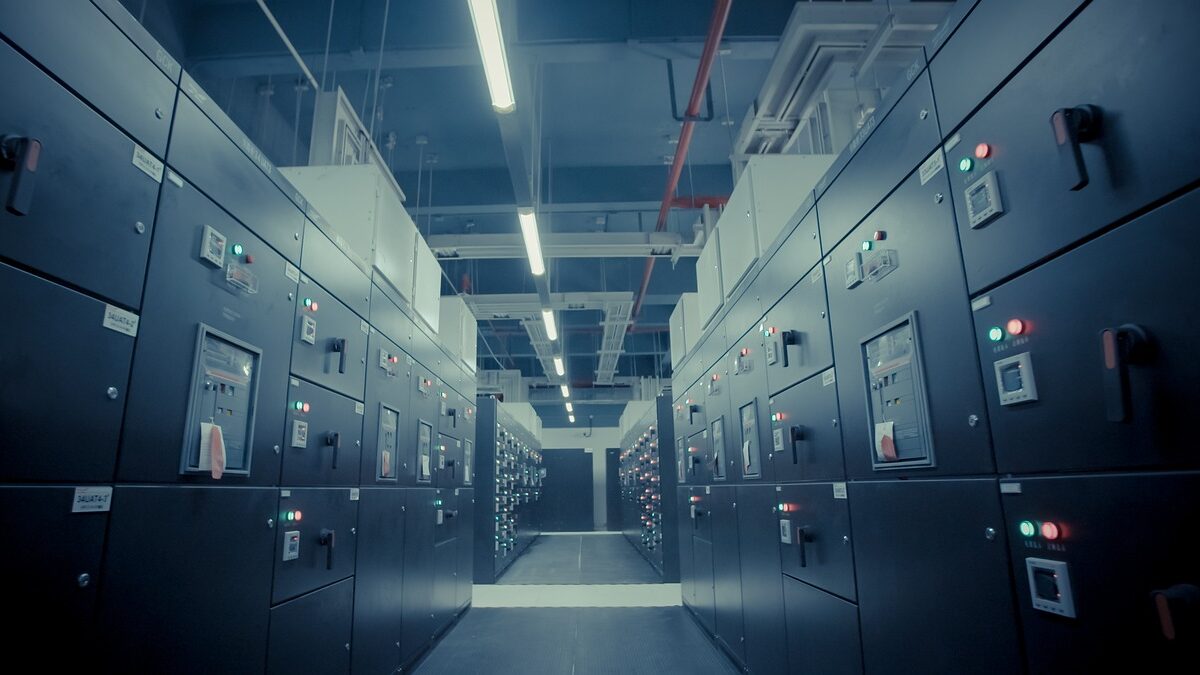
What Are Functions in MySQL
In MySQL, a function is a reusable block of code that performs a specific task and returns a value, Functions can accept parameters and are commonly used to encapsulate frequently used logic, such as calculations or data transformations.
How to create Functions with MySQL
To create a function in MySQL, you’ll need to follow these steps:
1. Choose a Function Name
Select a meaningful and descriptive name for your function, The name should reflect the purpose of the function to make your code more readable.
2. Define the Function
Use the ” CREATE FUNCTION
“ statement to define your function. Here’s the basic syntax :
CREATE FUNCTION function_name ( parameter1 datatype , parameter2 datatype, ... )
RETURNS return_datatype
BEGIN
-- Function logic here
END;
function_name
: Replace this with the chosen name for your function.parameter1
,parameter2
, …: Specify any input parameters your function needs.return_datatype
: Define the data type of the value that the function will return.
3. Write the Function Logic
Inside the BEGIN
and END
block, write the logic for your function. You can use SQL statements, control structures like IF
and CASE
, and any necessary calculations.
Here’s an example of a simple function that calculates the square of a number:
DELIMITER //
CREATE FUNCTION CalculateSquare(number INT)
RETURNS INT
BEGIN
DECLARE square INT;
SET square = number * number;
RETURN square;
END;
//
DELIMITER ;
4. Set the Delimiter
Before creating the function, you should set the delimiter to something other than the default semicolon “;” to prevent conflicts with the semicolons used within the function body. In the example above, we set the delimiter to //
.
5. Create the Function
Execute the “CREATE FUNCTION
“ statement with the new delimiter to create your function.
6. Use the Function
You can now use your user-defined function like any other built-in function in MySQL. For example:
SELECT CalculateSquare(5);
-- Returns 25
Conclusion
Creating user-defined functions in MySQL can help you streamline your database operations and make your code more modular and maintainable, By following the steps outlined in this guide, you can harness the power of custom functions to perform complex calculations and tasks within your MySQL database.
Pingback: How to create Stored Procedures in MySQL - The Best Coding